Gameplay Modules
1. Player(c++)
i. Movement
Basic movements: Walking, Crouching, Sprinting, Jumping
Advance movements : Sliding, Wallrun, Double Jump, Ledge Grab
All the advance movements are compatible with other. All the different movement can only be performed if they met a certain condition and each has its own walk speed, and all this functionality is being handled by a single function, which take a single parameter i.e., MovementToPerform and will return a bool depending on whether the player can perform this movement or not, and if it can it will also set the player walk speed to the new movement speed.
ii. Pickup System
The project is implemented with easy to use, well-optimized pickup system. And each pickup item has its own object channel and Pickup Interface as we don’t want to cast to each item. Currently it’s a dual weapon system means player can pickup only two items i.e., two guns and two throwables. All the guns are inherited from WeaponBase class and all the throwables are inherited from ThrowableBase.

2. Encounter Space(c++ and Blueprint)
-
Combat space where all the combat takes place.
-
Used by the AI to communicate with other AIs, but all the AIs must be inside it.
-
It stores all the cover objects, some selected items like doors( which will be closed/locked at the start of the combat and unlocked after the combat), and all the AI controllers at the beginning, so the controller can assign the cover to the AI, also if we spawn AI inside the encounter space, that AI will tell the encounter space then it entered the space so that it can be tracked by the space.
-
It keeps track of all living AI inside it, which when becomes 0, the player will be able to move to the next area or we can call for another enemy wave depending on the situation.
3. AI
i. Guard AI(Base AI class)(c++)
-
Cover ability
-
Sight, Hearing, Damage & Touch Sense
-
Communication with allies: During Combat, if the player is not visible to the AI, it will ask other Ais inside the space whether they can see the player or not.
-
Patrolling, Investigation & Combat Ability
-
Medium health/damage.
-
Weapon: Assault Rifle
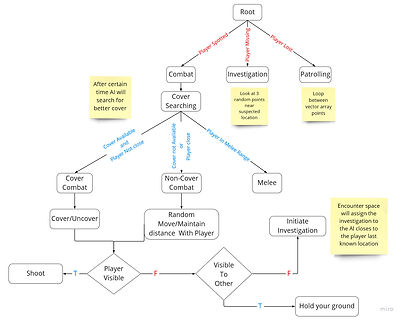
ii. Heavy AI(c++)
-
Sight, Hearing, Damage & Touch Sense
-
Has a special weapon ability( will shoot the shotgun at auto mode for specified ammo count)
-
High Health/Damage
-
Weapon: Shotgun
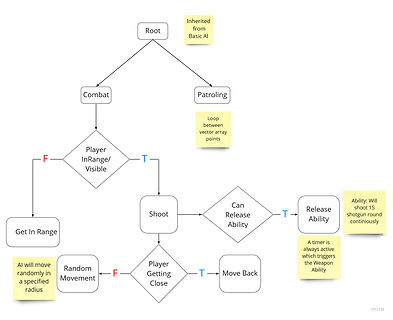
iii. MadDog(c++)
-
Basic chase and attack behavior
-
Hand throwing/recalling ability with player movement tracking
-
The damage for the melee is all handled in the anim montage through a damage notify class, a custom class that can be used with any skeletal mesh.
-
High Health/Damage

iv. Emo(Blueprint)
-
Basic roaming AI, with chase and explode behavior, as soon as the player gets in its radius it will start chasing him and when close will explode.
v. Wall Turret(Blueprint)
-
​Rare types of ai controlled fixed machine gun, that will follow the player and shoots heavy bullets for a specified time and then stops for certain seconds. This gun is can only we interacted by the player at certain levels only.
4. Weapons(c++)
-
Each gun/throwable is inherited from its own base class.
-
Overridable function for different functionality for specific weapons.
-
Contains a non-overridable function that handles, guns traces, animations, and ammo count.
-
The system is designed in such a way that we only need to change certain variables values inside bp, to change them from auto to semi-auto , or from single projectile to multiple.
-
Easy to create new weapons with basic functionality.
-
Weapons have their own collision channel to save some performance when looking for a pickable weapon
-
Shooting/Pickable guns/throwables derived from a single base class, and when picking up the gun, that weapon is not spawning again, it just attaches to the weapon socket on player mesh and for the throwables, we are saving its class and their count. In the case of throwables, we are spawning them when tossing them.
Level Screenshots
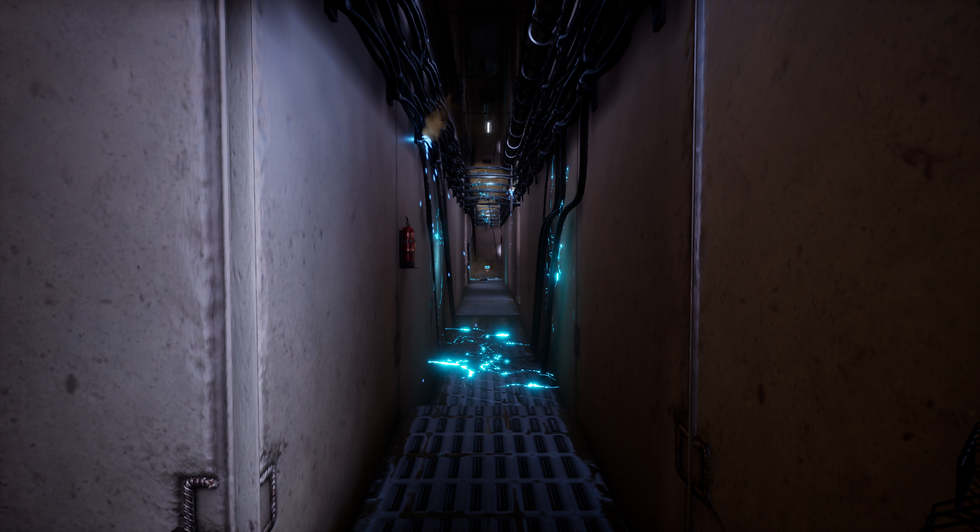
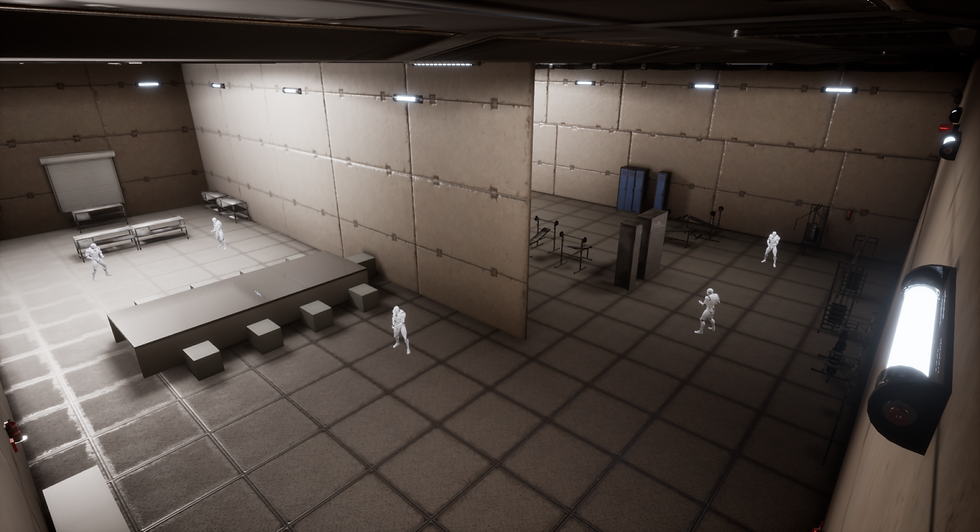
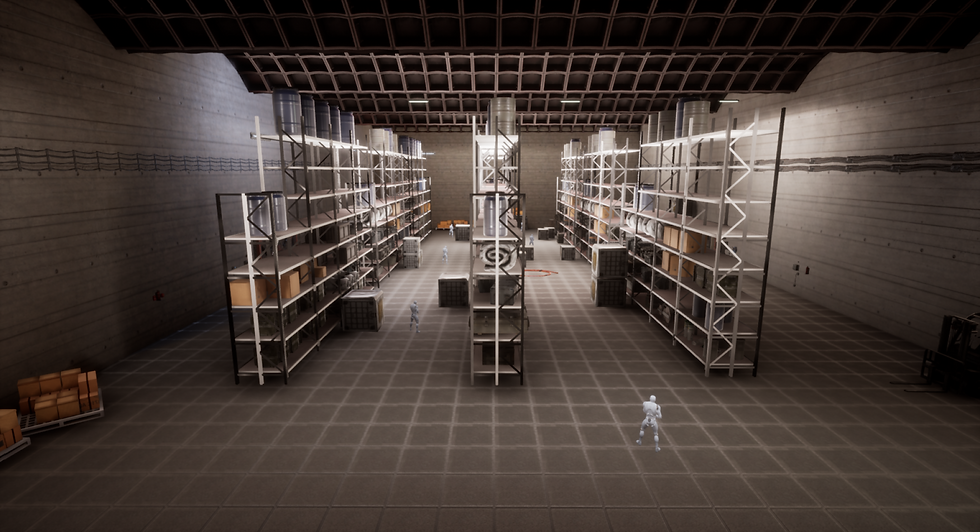
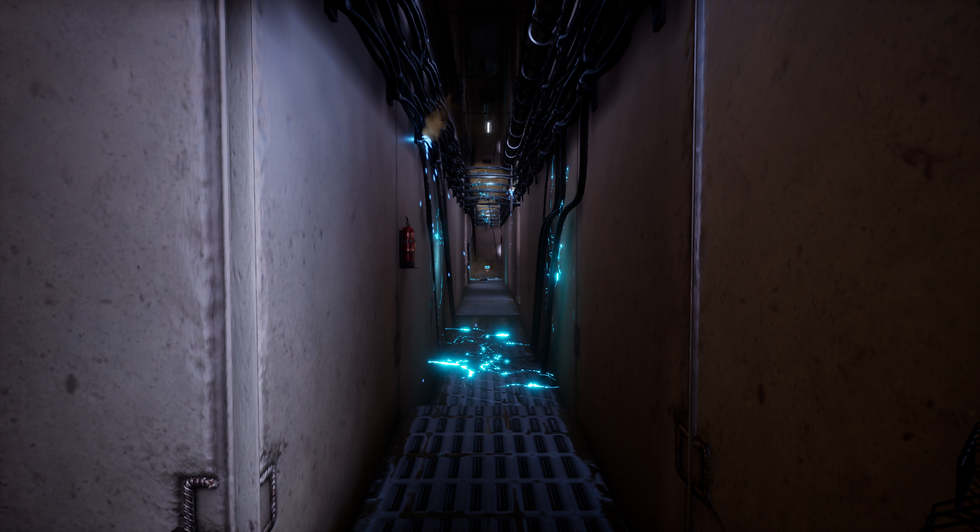